Enhancing SaaS Applications with AI-Powered Hybrid Search (FAISS + Elasticsearch)
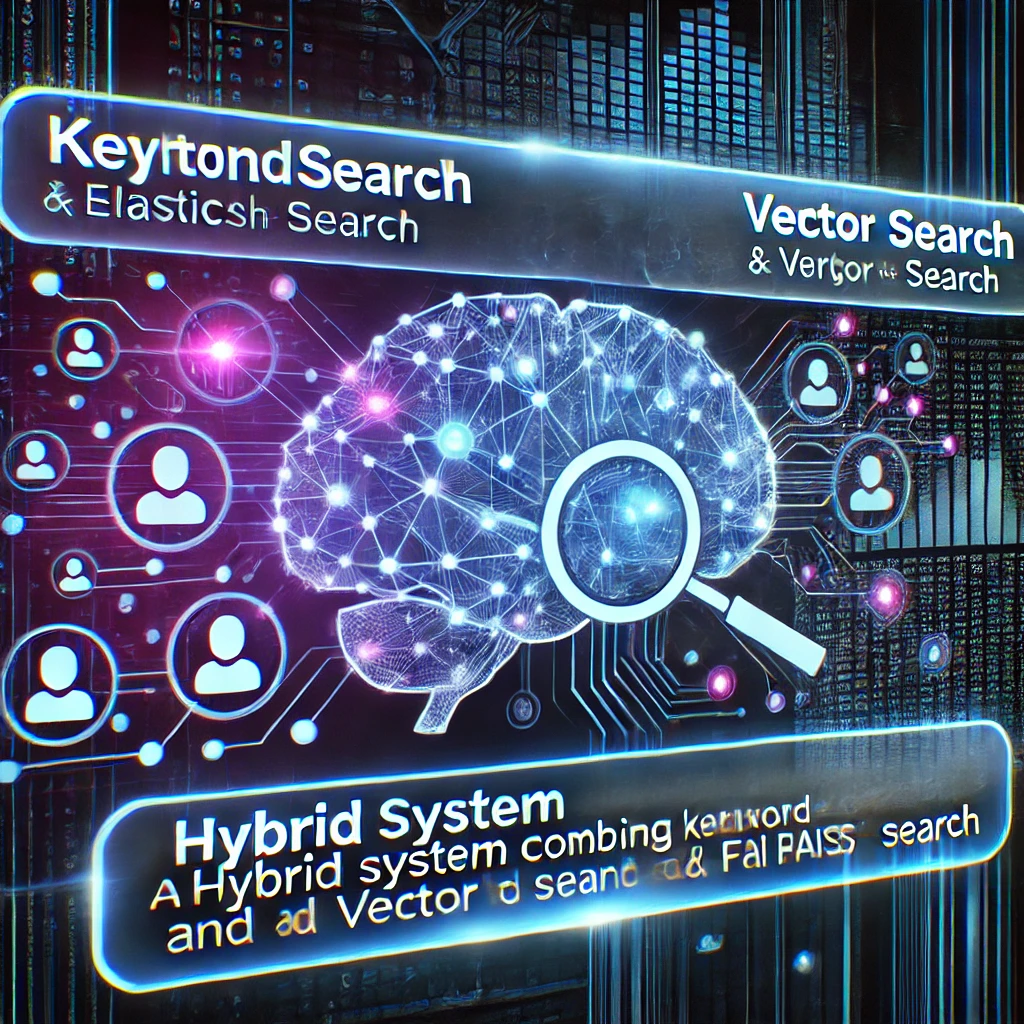
How to Build a Scalable, AI-Driven Search System for Your SaaS App
🔹 Introduction
Search is a core feature in SaaS applications, from e-commerce platforms to customer support portals. However, traditional keyword-based search engines like Elasticsearch struggle with:
• Understanding synonyms (e.g., “wireless headphones” vs. “Bluetooth headphones”).
• Matching user intent (e.g., searching for “comfortable running shoes” but getting unrelated results).
• Ranking results effectively (e.g., not showing the most relevant results at the top).
❌ Limitations of Keyword-Based Search
Traditional search engines use BM25 (a ranking algorithm based on term frequency) to rank results. However, these models rely on exact keyword matches, meaning:
✅ Great for structured data and exact keyword searches
❌ Fails when users phrase queries differently
❌ Ignores the context or meaning behind the words
To solve this, we need Hybrid Search, which combines semantic (AI-based) and lexical (keyword-based) search.
🔹 What is Hybrid Search?
Definition
Hybrid Search is an AI-powered search approach that combines:
1. Lexical Search (Keyword-Based Search)
• Uses Elasticsearch or OpenSearch for exact keyword matches.
• Works well for structured data and documents.
2. Semantic Search (Vector-Based Search)
• Uses FAISS (Facebook AI Similarity Search) or Pinecone for context-based retrieval.
• Converts words into numerical vectors to find similar meanings.
3. Re-Ranking (LLM-Based Re-Ranking)
• Uses an AI model (Cross-Encoder) to re-rank results based on query relevance.
🔹 What is Lexical Search?
Definition
Lexical Search (also known as Keyword-Based Search) retrieves documents by matching exact words from the query with the text in the dataset.
How It Works
• Uses Elasticsearch or OpenSearch to index text.
• Queries return results using BM25 (Best Matching 25) ranking.
• Results are fast and efficient but limited to exact matches.
Example:
A user searches for “Bluetooth headphones”, and Elasticsearch retrieves:
• “Bluetooth Headphones with Noise Cancellation”
• “Best Wireless Headphones”
However, it might miss “Wireless Earbuds” because “earbuds” ≠ “headphones” (no synonym handling).
Why Use Lexical Search?
✅ Fast & Efficient
✅ Supports Structured Queries (Filters, Categories, etc.)
❌ Fails When Synonyms or Different Wording Is Used
🔹 What is Semantic Search?
Definition
Semantic Search is an AI-driven search technique that understands the meaning of a query rather than just matching keywords.
For example:
• Searching for “wireless earbuds” should return “Bluetooth headphones” even though “Bluetooth” and “wireless” are different words.
• Searching for “budget laptop” should prioritize “affordable laptops” over “premium gaming laptops”.
How Does Semantic Search Work?
1. Convert text into vector embeddings
• AI models like BERT or Sentence Transformers transform words into high-dimensional numerical vectors.
2. Compare vectors using FAISS or Pinecone
• Instead of matching words, we compare vectors to find semantically similar results.
🔹 What is Vector Search?
Definition
Vector Search is a mathematical way of finding similar items by representing text as high-dimensional vectors.
Example of Vector Representation
Imagine the words “king”, “queen”, “man”, and “woman” as points in space:
• “King” – “Man” + “Woman” ≈ “Queen”
• The words “wireless” and “Bluetooth” might be closer than “Bluetooth” and “smartphone”.
How Does Vector Search Work?
• Text is encoded into embeddings (e.g., using Sentence Transformers).
• Embeddings are stored in FAISS (a high-speed vector database).
• Nearest Neighbor Search (ANN) finds the most relevant results.
🔹 Why Hybrid Search?
🔹 Combines Strengths of Both Approaches
• Keyword Search (Elasticsearch) → Finds exact word matches.
• Semantic Search (FAISS) → Finds contextually relevant results.
🔹 Improves User Experience
• Ensures better product discovery, knowledge base retrieval, and content recommendations.
🔹 Scales Well for SaaS Apps
• FAISS + Elasticsearch can support millions of documents with sub-second search speeds.
🛠 Building an AI-Powered Hybrid Search System
Now, let’s implement hybrid search with:
• Elasticsearch (Lexical Search)
• FAISS (Vector Search)
• Cross-Encoder (Re-Ranking with AI)
🔹 Step 1: Install Dependencies
pip install faiss-cpu opensearch-py sentence-transformers numpy pandas
(Use faiss-gpu instead of faiss-cpu if you have a GPU for acceleration.)
🔹 Step 2: Generate Sample Product Data
products = [ {"title": "Wireless Headphones", "description": "Noise-canceling Bluetooth headphones", "category": "Electronics"}, {"title": "Running Shoes", "description": "Lightweight sports shoes for jogging", "category": "Footwear"}, {"title": "Smartphone", "description": "Latest Android phone with OLED display", "category": "Electronics"}, {"title": "Gaming Laptop", "description": "Powerful gaming laptop with high-end GPU", "category": "Computers"} ]
🔹 Step 3: Initialize FAISS for Vector Search
import faiss from sentence_transformers import SentenceTransformer import numpy as np embedding_model = SentenceTransformer("all-MiniLM-L6-v2") # Generate vector embeddings for products product_texts = [p["title"] + " " + p["description"] for p in products] product_vectors = embedding_model.encode(product_texts, normalize_embeddings=True) # Initialize FAISS Index dim = product_vectors.shape[1] index = faiss.IndexFlatL2(dim) index.add(np.array(product_vectors))
🔹 Step 4: Initialize Elasticsearch for Keyword Search
from opensearchpy import OpenSearch es_client = OpenSearch(hosts=[{"host": "localhost", "port": 9200}], http_auth=("elastic", "changeme")) index_name = "products"
Insert Data into Elasticsearch
for i, product in enumerate(products): es_client.index(index=index_name, id=i, body=product)
🔹 Step 5: Implement Hybrid Search
def hybrid_search(query, top_k=5):
# Lexical Search (Elasticsearch)
query_body = {"query": {"multi_match": {"query": query, "fields": ["title", "description"]}}}
es_response = es_client.search(index=index_name, body=query_body, size=top_k)
lexical_results = [hit["_source"] for hit in es_response["hits"]["hits"]]
# Semantic Search (FAISS)
query_vector = embedding_model.encode([query], normalize_embeddings=True)
D, I = index.search(np.array(query_vector), k=top_k)
semantic_results = [products[i] for i in I[0]]
# Merge Results
results = {p["title"]: p for p in semantic_results}
for doc in lexical_results:
results[doc["title"]] = doc
return list(results.values())[:top_k]
🚀 Conclusion
Hybrid Search boosts search accuracy and improves user experience in SaaS applications. Using Elasticsearch, FAISS, and AI re-ranking, you can deliver highly relevant results.
Would you like to integrate this AI search into your SaaS app? 🚀🔥 Get in touch now and take your SaaS app to the next level! 🚀
#AI #MachineLearning #HybridSearch #SemanticSearch #VectorSearch #SoftwareEngineering #SaaS #Elasticsearch #FAISS #NLP
Leave a Reply